- About Us
- Services
Ready to start your dream project?
- Domain Expertise
- Case Study
- Blogs
- Careers
- Contact Us
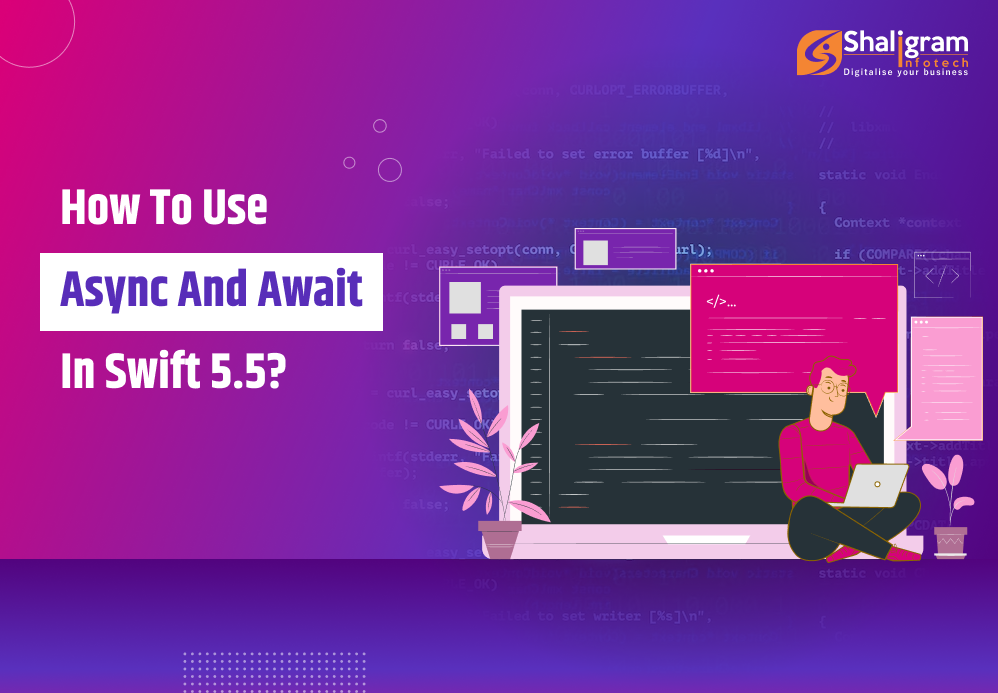
When the Swift 5.5 version was released in 2021, Apple introduced the async function with it. Similar to JavaScript type other programming languages, the async function makes it easy for developers to run complex tasks. This means there is no need to wait for the execution of the first code to start the second one.
With the help of async/await we can call more than one function at the same time. It lets you have a better code structure, less pressure on the main thread, and less waiting for the user to load the data on the screen.
Async/Await
Async says any function with asynchronous words provides independent execution and does not disturb any other program.
Await says that async does not listen to anyone except me. This means we have to request await to call an async method. We do that by adding the “await” keyword before calling an async method.
Async Keyword
Now we know what an async keyword means and how async methods work. So now let’s see an example with code.
func method1() async { //Do Something. } func method2() async { //Do Something. } |
This is how the “async” keyword is used to create an Asynchronous method.
Await Keyword
So now that we have created asynchronous methods, we will have to call them from where we want to execute that method. Here we will be using the “await” keyword to call an asynchronous method.
await method1() await method2() |
However, calling the asynchronous method this way is not going to be easy. We will need an asynchronous environment to call them.
let’s see how it’s done.
From the Swift 5.6 version, support for async was given to TaskGroup operations. This means from now on, we have to use Task. init to call asynchronous methods instead of using async(operations:).
To start using asynchronous methods in non async environment, code something like shown below:
Task.init { await method1() await method2() } |
Now the methods created with an async keyword as shown above will be called from the body of TaskInit with awaiting.
Async/Await v/s Trailing closure
Let’s say we need some value from method one to execute the second one.
Before having the async/await feature, we used to pass the value in trailing closure and send it to the second one through the parameter as shown below:
//Declaration func method1(_ completion: @escaping (String) -> Void) { //Do Something. completion(“value”) } func method2(_ name: String) { //Do Something. } //Execution method1 { name in self.method2(name) } |
Now with the help of async/await, we can directly get the value from the first method, await for that value, and then continue the execution.
//Declaration func method1() async -> String { //Do Something. return “Value” } func method2() async { let name = await method1() print(name) } //Execution await method2()
|
Conclusion
In this blog, we saw, what the async/await feature is, why it should be used, and what we used to do before having it.
Async/await is good for our processing chips. Here every asynchronous method separates its thread. So, the main thread does not get so much pressure. Moreover, it saves time for users that was otherwise spent waiting for any method to complete its execution.